I’m glad you brought this up, since it’s a more subtle point when building subs.
Rule of thumb: make all parameters Literals (vs. Variables) UNLESS you need the value to change, e.g., the subroutine is calculating/returning a value.
Here’s an example with this GetLengthOfMonth sub where I needed it to tell me the number of days in a particular month so I pass which month it is, which year, and I want to get back my answer of: 28,29,30 or 31.
Note (highlighted in blue) the DaysInMonth is the value being returned. (In computer science lingo, we called this passed by reference vs. passed by value.)
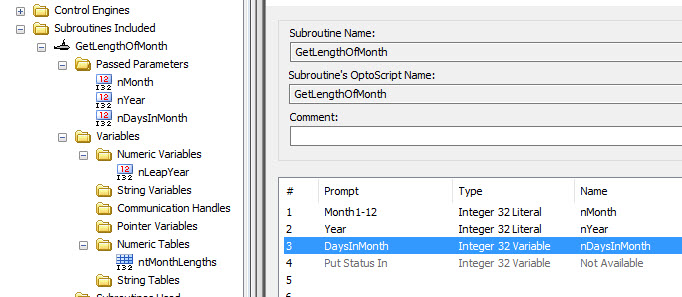
This also means, you have more choices when CALLING the subroutine. For example, if I wanted to call this sub to see if this year is a leap year, I could just do this:
GetLengthOfMonth( 2, 2017, nDaysInFeb );
or something like this:
GetLengthOfMonth( nThisMonth, nThisYear, nDaysInFeb );
Note in the first one I’m passing literals (the 2 and the 2017). If I’d made those first two subroutine parameters variables instead of literals, I wouldn’t have a choice, my only option would be to pass in varibles as shown in the second example.
That’s why you want the more flexible option of the Literal (the number or variable is like literal, unchanging, once it gets into the sub) whenever possible. You’re also less likely to inadvertently change something you didn’t mean to change.
I hope that helps!
-OptoMary